Persistent storage for your android application can benefit you in many ways. Some of the common use cases could be using it for storing some relevant pieces of data which can be accessed even when the device cannot access the network. In this article, we will learn about the Room persistence library and its primary components.
What is Room?
Room is a persistence library provided by Android that is used as an abstraction layer over SQLite. It makes use of the full potential of SQLite while enabling more robust database access.
Some benefits of Room are -
- Verifies SQL queries at compile time.
- Convenience annotations that reduce repetitive and error-prone boilerplate code.
- Streamlined database migration paths.
Primary Components
The primary components of Room library are -
- Database class
- Data Entities
- DAOs (Data Access Objects)
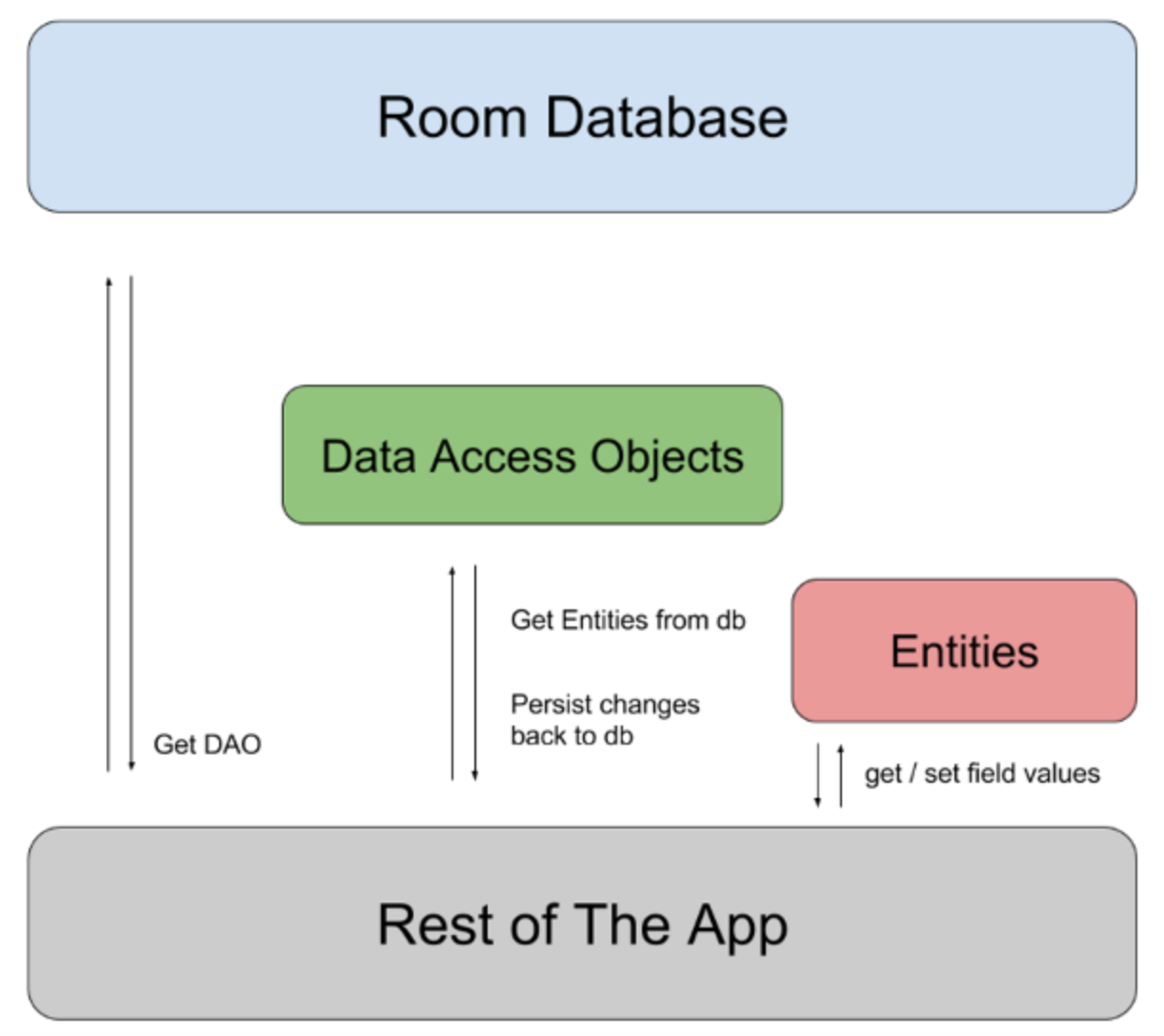
Let's have a look at each one of these in more detail.
- Database Class -
It holds your database and acts as the main access point to the database. It also provides the DAOs that the application can use to access the corresponding table.
DB class is annotated with @Database
and includes the list of all entities that are part of the database. This class must extend RoomDatabase
.
All the examples are explained by taking an example of User
entity.
2. Data Entities
Entities represent tables for your app's database. They are defined by annotating a data class with @Entity
. Entity includes fields for each column in the corresponding table in the database.
Let's define an entity User
with fields - id
, firstName
and lastName
Composite primary keys can be defined as follows -
Ignoring fields - Fields that should not be added as columns to the table can be ignored with @Ignore
.
3. DAOs (Data Access Objects)
They provide methods that are used by your app to query, insert, delete or update data in the database.
It can be an interface or an abstract class. At compile time, Room automatically generates implementation for DAO. It must be annotated with @Dao
. DAOs don’t have properties, but they have one or more functions that interact with the data in your app’s database.
Let's define a UserDao
-
This UserDao
contains 4 methods
insertAll
- annotated by@Insert
to insert users into DB.delete
- annotated by@Delete
to delete the user from DB.getAll
- annotated by@Query
to query all users from DB.getUserById
- annotated by@Query
to get a single user with a matchingid
from DB.
DAO methods are classified into two types -
- Convenience methods - These methods let you insert, update and delete without writing SQL code. Eg -
Insert
,Delete
andUpdate
. - Query methods - These are custom SQL queries to interact with the database. Eg -
Query
.
Room also has onConflictStrategy
support for handling conflict in queries.
You can choose any of the following options in case of conflict -
- ABORT - abort the transaction
- FAIL - fail the transaction
- IGNORE - ignore conflict and continue
- REPLACE - replace old data and continue
- ROLLBACK - rollback the transaction
Summing up, Room allows you to quickly and easily extract all SQLite features, which will save you a tonne of time.
That's it from this article. Stay tuned for more such content.